Value Type vs. Reference Type
A Reference Type stores the memory address of where the value is stored.

string name = "Stew Dent";
Reference Types - null
null
refers to memory address zero (which doesn't exist)
Die fuzzyRedDie = null;
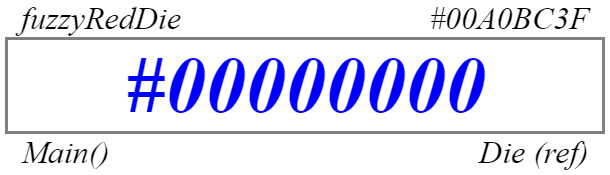
Reference Types - Instance
new
instantiates an object based on the data type
fuzzyRedDie = new Die(20);
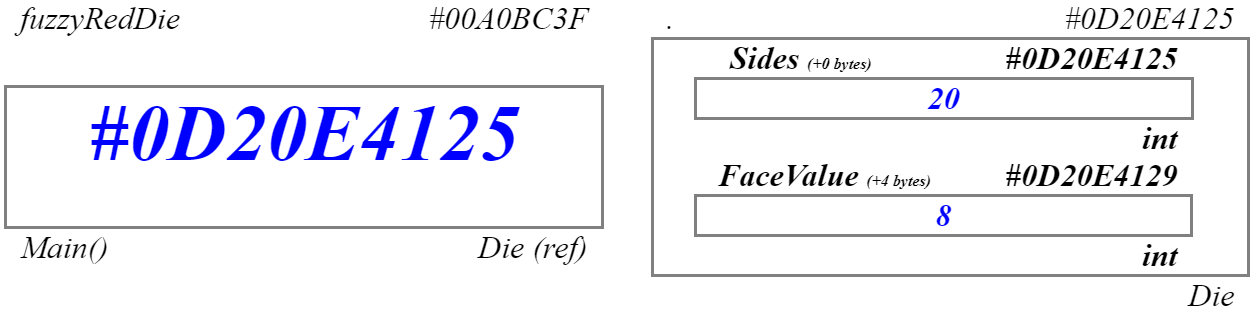
Rules for Variables/Values/Data Types
The following rules are applied to all variables, values and data types.
- Any value stored in a variable must be of the same data type as the variable.
- Variables must be declared before they can be used.
- A variable's data type can only be declared once.
- Each variable can only hold one value at a given time.
- Each variable must have a distinct name.